多态性这个词的意思是有多种形式。简单地说,我们可以将多态性定义为一条消息以多种形式显示的能力。一个现实生活中的多态性例子,一个人在同一时间可以有不同的特征。像男人一样,同时也是父亲、丈夫和雇员。所以同一个人在不同的情况下有不同的行为。这叫做多态性。多态性被认为是面向对象编程的重要特征之一。
null
C++中的多态性主要分为两类:
- 编译时多态性
- 运行时多态性
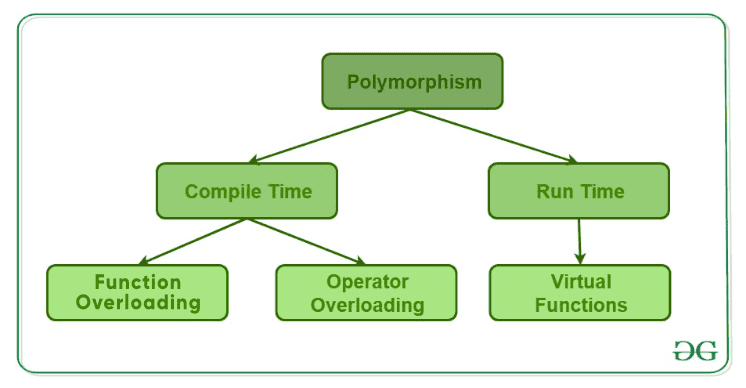
多态类型
- 编译时多态性 :这种多态性是通过函数重载或运算符重载实现的。
- 函数得载 :当有多个同名但参数不同的函数时,这些函数称为 超载 .函数可以通过 参数数量的变化 或/和 参数类型的变化 . 函数重载规则
// C++ program for function overloading
#include <bits/stdc++.h>
using
namespace
std;
class
Geeks
{
public
:
// function with 1 int parameter
void
func(
int
x)
{
cout <<
"value of x is "
<< x << endl;
}
// function with same name but 1 double parameter
void
func(
double
x)
{
cout <<
"value of x is "
<< x << endl;
}
// function with same name and 2 int parameters
void
func(
int
x,
int
y)
{
cout <<
"value of x and y is "
<< x <<
", "
<< y << endl;
}
};
int
main() {
Geeks obj1;
// Which function is called will depend on the parameters passed
// The first 'func' is called
obj1.func(7);
// The second 'func' is called
obj1.func(9.132);
// The third 'func' is called
obj1.func(85,64);
return
0;
}
输出:
value of x is 7 value of x is 9.132 value of x and y is 85, 64
在上面的示例中,一个名为 func 在三种不同的情况下表现不同,这是多态性的特点。
- 运算符重载 C++还提供了对过载操作符的选项。例如,我们可以使string类的运算符(“+”)连接两个字符串。我们知道这是加法运算符,其任务是将两个操作数相加。因此,在整数操作数之间加一个运算符“+”,在字符串操作数之间连接它们。 实例 :
// CPP program to illustrate
// Operator Overloading
#include<iostream>
using
namespace
std;
class
Complex {
private
:
int
real, imag;
public
:
Complex(
int
r = 0,
int
i =0) {real = r; imag = i;}
// This is automatically called when '+' is used with
// between two Complex objects
Complex operator + (Complex
const
&obj) {
Complex res;
res.real = real + obj.real;
res.imag = imag + obj.imag;
return
res;
}
void
print() { cout << real <<
" + i"
<< imag << endl; }
};
int
main()
{
Complex c1(10, 5), c2(2, 4);
Complex c3 = c1 + c2;
// An example call to "operator+"
c3.print();
}
输出:
12 + i9
在上面的示例中,运算符“+”重载。运算符“+”是一个加法运算符,可以将两个数(整数或浮点)相加,但这里的运算符用于执行两个虚数或复数的加法。要详细了解运算符重载,请访问 这 链接
- 函数得载 :当有多个同名但参数不同的函数时,这些函数称为 超载 .函数可以通过 参数数量的变化 或/和 参数类型的变化 . 函数重载规则
- 运行时多态性 :这种多态性是通过函数重写实现的。
- 函数重写 另一方面,当派生类定义了基类的一个成员函数时,就会发生这种情况。这个基函数被称为 压倒 .
// C++ program for function overriding
#include <bits/stdc++.h>
using
namespace
std;
class
base
{
public
:
virtual
void
print ()
{ cout<<
"print base class"
<<endl; }
void
show ()
{ cout<<
"show base class"
<<endl; }
};
class
derived:
public
base
{
public
:
void
print ()
//print () is already virtual function in derived class, we could also declared as virtual void print () explicitly
{ cout<<
"print derived class"
<<endl; }
void
show ()
{ cout<<
"show derived class"
<<endl; }
};
//main function
int
main()
{
base *bptr;
derived d;
bptr = &d;
//virtual function, binded at runtime (Runtime polymorphism)
bptr->print();
// Non-virtual function, binded at compile time
bptr->show();
return
0;
}
输出:
print derived class show base class
要详细了解运行时多态性,请访问 这 链接
- 函数重写 另一方面,当派生类定义了基类的一个成员函数时,就会发生这种情况。这个基函数被称为 压倒 .
本文由 严酷的阿加瓦尔 .如果你喜欢GeekSforgek,并想贡献自己的力量,你也可以使用 写极客。组织 或者把你的文章寄去评论-team@geeksforgeeks.org.看到你的文章出现在Geeksforgeks主页上,并帮助其他极客。
如果您发现任何不正确的地方,或者您想分享有关上述主题的更多信息,请写下评论。
© 版权声明
文章版权归作者所有,未经允许请勿转载。
THE END